Main() without Class (and it's not Kotlin)
Jdk 21 has just reached Rampdown Phase One and will be released with some awesome features such as Virtual Threads, sequenced collections, generational ZGC, and pattern matching. As if those aren’t enough, Java is becoming more accessible to new programmers thanks to the JEP (Java Enhancement Proposal) 445: Unnamed Classes and Instance Main Methods.
In this short tutorial, we’ll take a look at He-Who-Must-Not-Be-Named
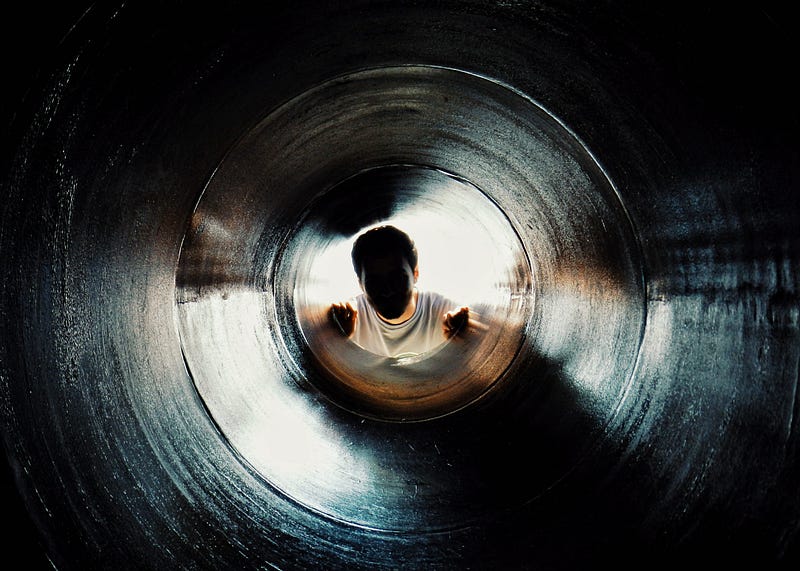
Photo by Micaela Parente on Unspash
Imagine learning the first concepts of Java without the burden of learning all the boilerplate needed to run a simple script that does the sum of two numbers. Thanks to this brand-new enhancement, It’s now possible to write:
void main() {
int a = 1;
int b = 2;
int c = a + b;
}
Instead of the draconian:
public class MainClass {
public static void main(String[] args) {
int a = 1;
int b = 2;
int c = a + b;
}
}
But what does that main() method standing alone means? Where does it reside? Is it possible to reference it from outside? First of all, let’s make a distinction between anonymous classes ad unnamed classes. I think It’s necessary considering how close these words are.
Anonymous classes are a particular type of NESTED class used when we need to create a new class and reference it only once. A typical example we all have seen is with Runnables:
// Guess what, I have a name but I'm anonymous
Runnable action = new Runnable() {
@Override
public void run() {
System.out.println("RUN Forrest, RUN!!");
}
};
An unnamed class instead is a class that “simply” is not possible to reference by name from any other Class. Our main method will be in fact placed in an unnamed class, in an unnamed package, in an unnamed module.
The unnamed class will be only triggered if we run the program, so it has to contain a main method. Additionally, as a consequence of being unnamed, it’s impossible to extend it, it can’t implement interfaces, can’t define constructors, etc. Probably it’s even an overkill calling it a class. Should be named unnamed script, really.
Let’s see a bigger example (remember to eventually run this code with the ”–enable-preview” flag):
int zero = 0;
int oneHundred = 100;
int aNumber = 1234554321;
void main() {
assert xxxx(zero);
assert !xxxx(oneHundred);
assert xxxx(aNumber);
}
boolean xxxx(int x) {
if(x < 0) {
return false;
}
if(x < 10) {
return true;
}
String y = String.valueOf(x);
int half = y.length() / 2;
for(int i=0; i < half; i++) {
if(y.charAt(i) != y.charAt(y.length()-1-i)){
return false;
}
}
return true;
}
It’s scripting made simple, with Java! This will be pretty awesome for testing with DataStructure and Algorithm as well. Just look at how many public static void main(String[] args)) I had to write!
PS: While this seems to be a further step towards the “free functions” (functions outside classes) that Kotlin provides, this is completely outside the original idea of this JEP. If you feel depressed now, please remember that we Java programmers, do not need Kotlin. We have the Static keyword that makes us able to evade the burdens of Object-Oriented Programming by bypassing the concept of Class entirely, accessing a function without the need for a specific containing structure. The class, in this case, is simply the “namespace”.
PS2: How would you call that “xxxx” method up there? :)
Thank you for reading!